During a penetration test, there may be instances where you need to develop a tool that is lightweight and quiet. After conducting reconnaissance on the system, you can obtain a clearer understanding of how the network’s systems are structured. Armed with this knowledge, you can create a tool that performs multiple, low-impact tasks. In this article I will demonstrate how to construct a Python script that incorporates a command line utility that manages Linux systems through the use of Ansible.
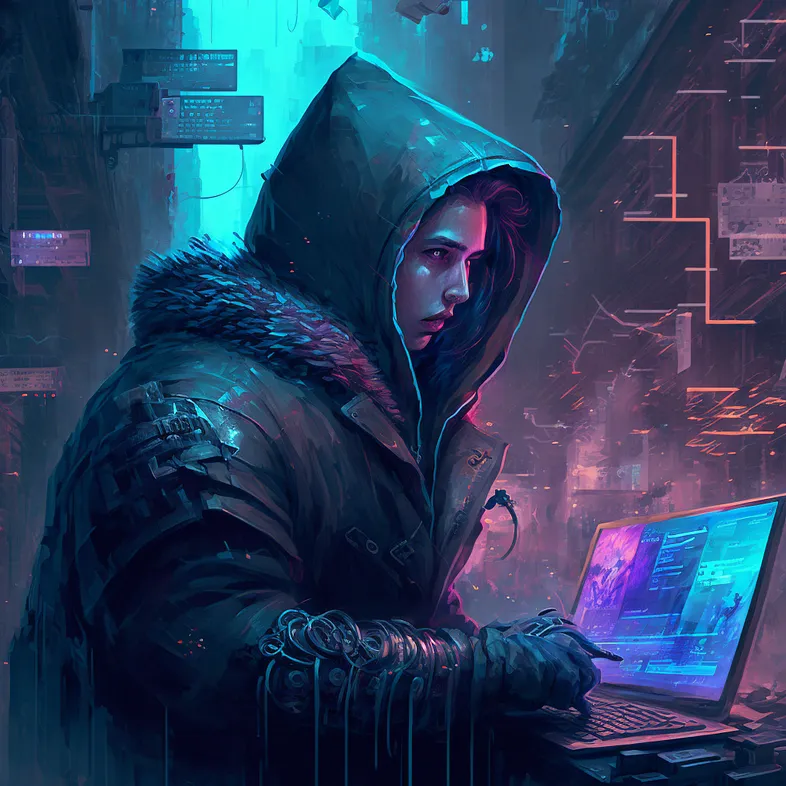
We will need to import the os
and subprocess
modules to interact with the operating system and execute commands.
import os
import subprocess
Now I can build out the functions. For this I want five functions. The first function will facilitate the installation of Ansible, while the second function will enable the addition of my SSH key to a remote server. The third function will allow the searching of the system for file types, and the fourth function will enable the downloading of files and folders from multiple servers. The fifth function will facilitate the transfer of files between servers. Additionally, we will include a function, denoted as 0, to exit the script.
The first function gives the option to install Ansible on the system using the subprocess.run
function to execute the necessary commands.
def install_ansible():
subprocess.run(["sudo", "apt", "update"])
subprocess.run(["sudo", "apt", "install", "software-properties-common"])
subprocess.run(["sudo", "apt-add-repository", "--yes", "--update", "ppa:ansible/ansible"])
subprocess.run(["sudo", "apt", "install", "ansible"])
The second function prompts the user for the IP address of the remote server, the username on the remote server, and the path to the SSH key. It then executes the ssh-copy-id
command using subprocess.run
to add the SSH key to the remote server.
def add_ssh_key():
ip = input("Enter the IP address of the remote server: ")
username = input("Enter the username on the remote server: ")
ssh_key_path = input("Enter the path to the SSH key: ")
subprocess.run(["ssh-copy-id", f"{username}@{ip}", "-i", ssh_key_path])
The third function prompts the user for the IP addresses of the systems to scan and the file types to scan for. It then uses a loop to execute the ansible
command with the find
module to scan each system for the specified file types.
def download_files():
ips = input("Enter the IP addresses of the systems to download files from (comma-separated): ")
files = input("Enter the files to download (comma-separated): ")
dest = input("Enter the destination folder to save the files: ")
for ip in ips.split(","):
for file in files.split(","):
subprocess.run(["ansible", f"{ip}", "-m", "fetch", "-a", f"src={file} dest={os.path.join(dest, ip)}"])
The fourth function prompts the user for the IP addresses of the systems to download files from, the files to download, and the destination folder to save the files. It then uses nested loops to execute the ansible
command with the fetch
module to download each file from each system and save it to the specified destination folder.
def download_files():
ips = input("Enter the IP addresses of the systems to download files from (comma-separated): ")
files = input("Enter the files to download (comma-separated): ")
dest = input("Enter the destination folder to save the files: ")
for ip in ips.split(","):
for file in files.split(","):
subprocess.run(["ansible", f"{ip}", "-m", "fetch", "-a", f"src={file} dest={os.path.join(dest, ip)}"])
The fifth function prompts the user for the IP address of the source server, the IP address of the destination server, and the files to transfer. It then uses a loop to execute the ansible
command with the copy
module to transfer each file from the source server to the destination server.
def transfer_files():
source_ip = input("Enter the IP address of the source server: ")
dest_ip = input("Enter the IP address of the destination server: ")
files = input("Enter the files to transfer (comma-separated): ")
for file in files.split(","):
subprocess.run(["ansible", f"{source_ip}", "-m", "copy", "-a", f"src={file} dest={dest_ip}:"])
To create a numerical list in the CLI we will need to define the main() function. This will display the menu by printing the list of options. We can then make a choice and execute the appropriate function based on the numerical input. If the user enters an invalid choice, the program displays an error message and returns to the main menu.
choice = input("Enter your choice: ")
if choice == "1":
install_ansible()
elif choice == "2":
add_ssh_key()
elif choice == "3":
scan_files()
elif choice == "4":
download_files()
elif choice == "5":
transfer_files()
elif choice == "0":
break
else:
print("Invalid choice")
This final section of the script is a standard Python idiom that checks if the script is being run as the main program. If it is, the main()
function is called to start the program. If it’s not, the script is being imported as a module and the main()
function is not executed.
if __name__ == "__main__":
main()
The response will look like the following

There you have it. You now have the ability to construct any tool you desire! Clap, follow, and share if you like this article and Happy Hunting!